How to Delete a File or Folder in Python
Deleting a file in Python is fairly easy to do. Let's discuss two methods to accomplish this task using different Python modules.
Using the 'os' Module
The os
module in Python provides a method called os.remove()
that can be used to delete a file. Here's a simple example:
import os
# specify the file name
file_name = "test_file.txt"
# delete the file
os.remove(file_name)
In the above example, we first import the os
module. Then, we specify the name of the file to be deleted. Finally, we call os.remove()
with the file name as the parameter to delete the file.
Note: The os.remove()
function can only delete files, not directories. If you try to delete a directory using this function, you'll get a IsADirectoryError
.
Using the 'shutil' Module
The shutil
module, short for "shell utilities", also provides a method to delete files - shutil.rmtree()
. But why use shutil
when os
can do the job? Well, shutil
can delete a whole directory tree (i.e., a directory and all its subdirectories). Let's see how to delete a file with shutil
.
import shutil
# specify the file name
file_name = "test_file.txt"
# delete the file
shutil.rmtree(file_name)
The code looks pretty similar to the os
example, right? That's one of the great parts of Python's design - consistency across modules. However, remember that shutil.rmtree()
is more powerful and can remove non-empty directories as well, which we'll look at more closely in a later section.
Deleting a Folder in Python
Moving on to the topic of directory deletion, we can again use the os
and shutil
modules to accomplish this task. Here we'll explore both methods.
Using the 'os' Module
The os
module in Python provides a method called os.rmdir()
that allows us to delete an empty directory. Here's how you can use it:
import os
# specify the directory you want to delete
folder_path = "/path/to/your/directory"
# delete the directory
os.rmdir(folder_path)
The os.rmdir()
method only deletes empty directories. If the directory is not empty, you'll encounter an OSError: [Errno 39] Directory not empty
error.
Using the 'shutil' Module
In case you want to delete a directory that's not empty, you can use the shutil.rmtree()
method from the shutil
module.
import shutil
# specify the directory you want to delete
folder_path = "/path/to/your/directory"
# delete the directory
shutil.rmtree(folder_path)
The shutil.rmtree()
method deletes a directory and all its contents, so use it cautiously!
Wait! Always double-check the directory path before running the deletion code. You don't want to accidentally delete important files or directories!
Common Errors
When dealing with file and directory operations in Python, it's common to encounter a few specific errors. Understanding these errors is important to handling them gracefully and ensuring your code continues to run smoothly.
PermissionError: [Errno 13] Permission denied
One common error you might encounter when trying to delete a file or folder is the PermissionError: [Errno 13] Permission denied
. This error occurs when you attempt to delete a file or folder that your Python script doesn't have the necessary permissions for.
Here's an example of what this might look like:
import os
try:
os.remove("/root/test.txt")
except PermissionError:
print("Permission denied")
In this example, we're trying to delete a file in the root directory, which generally requires administrative privileges. When run, this code will output Permission denied
.
To avoid this error, ensure your script has the necessary permissions to perform the operation. This might involve running your script as an administrator, or modifying the permissions of the file or folder you're trying to delete.
FileNotFoundError: [Errno 2] No such file or directory
Another common error is the FileNotFoundError: [Errno 2] No such file or directory
. This error is thrown when you attempt to delete a file or folder that doesn't exist.
Here's how this might look:
import os
try:
os.remove("nonexistent_file.txt")
except FileNotFoundError:
print("File not found")
In this example, we're trying to delete a file that doesn't exist, so Python throws a FileNotFoundError
.
To avoid this, you can check if the file or folder exists before trying to delete it, like so:
import os
if os.path.exists("test.txt"):
os.remove("test.txt")
else:
print("File not found")
OSError: [Errno 39] Directory not empty
The OSError: [Errno 39] Directory not empty
error occurs when you try to delete a directory that's not empty using os.rmdir()
.
For instance:
import os
try:
os.rmdir("my_directory")
except OSError:
print("Directory not empty")
This error can be avoided by ensuring the directory is empty before trying to delete it, or by using shutil.rmtree()
, which can delete a directory and all its contents:
import shutil
shutil.rmtree("my_directory")
Similar Solutions and Use-Cases
Python's file and directory deletion capabilities can be applied in a variety of use-cases beyond simply deleting individual files or folders.
Deleting Files with Specific Extensions
Imagine you have a directory full of files, and you need to delete only those with a specific file extension, say .txt
. Python, with its versatile libraries, can help you do this with ease. The os
and glob
modules are your friends here.
import os
import glob
# Specify the file extension
extension = "*.txt"
# Specify the directory
directory = "/path/to/directory/"
# Combine the directory with the extension
files = os.path.join(directory, extension)
# Loop over the files and delete them
for file in glob.glob(files):
os.remove(file)
This script will delete all .txt
files in the specified directory. The glob
module is used to retrieve files/pathnames matching a specified pattern. Here, the pattern is all files ending with .txt
.
Deleting Empty Directories
Have you ever found yourself with a bunch of empty directories that you want to get rid of? Python's os
module can help you here as well.
import os
# Specify the directory
directory = "/path/to/directory/"
# Use listdir() to check if directory is empty
if not os.listdir(directory):
os.rmdir(directory)
The os.listdir(directory)
function returns a list containing the names of the entries in the directory given by path. If the list is empty, it means the directory is empty, and we can safely delete it using os.rmdir(directory)
.
Note: os.rmdir(directory)
can only delete empty directories. If the directory is not empty, you'll get an OSError: [Errno 39] Directory not empty
error.
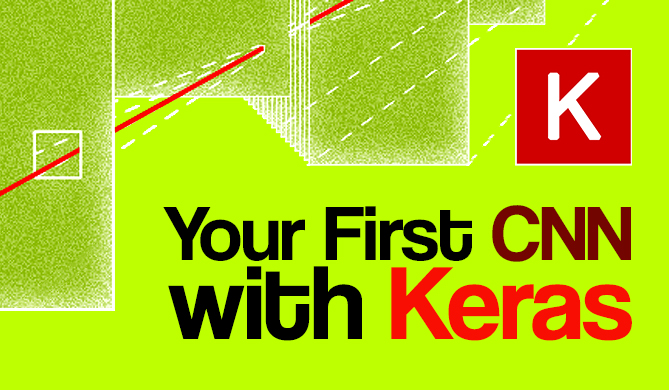
Building Your First Convolutional Neural Network With Keras
# python# artificial intelligence# machine learning# tensorflowMost resources start with pristine datasets, start at importing and finish at validation. There's much more to know. Why was a class predicted? Where was...
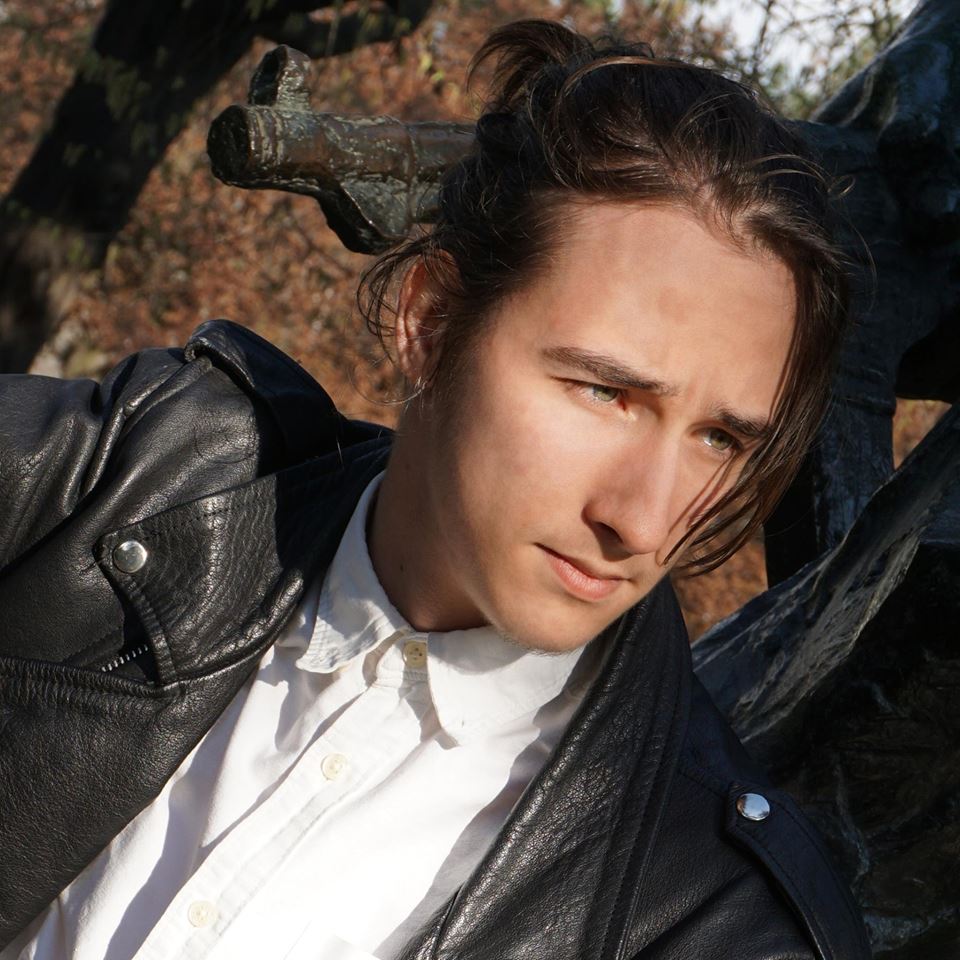